Scaling¶
Description¶
Scales base’s geometry with respect to a factor and an origin location.
Parameters¶
- base:
Base
The input base to be scaled.
- base:
- coordinates: list(str), default= None
The variable names that define the set of coordinates. If None, the default coordinate system variables is used. The coordinate system must be the Cartesian coordinate system.
- factor: class:seq(float)
The scaling factor for each of the axis of the base.
- origin: class:seq(float), default= None
Point used as reference for the scaling. If None, the origin of the coordinate system is used.
- memory_mode: bool, default= False
If True, the modifications are done directly on the input base to limit memory usage. If False, a new base is created.
Preconditions¶
The base must be in the Cartesian system of coordinates
Postconditions¶
If memory_mode = False, the input base remains unchanged and the output base has the same structure as the input base. If memory_mode = True, the input base is modified in-place and the returned base is the same object as the input base.
Example¶
The following example shows how to scale a base by a factor of 2 in the x direction, by a factor 0.6 in the y direction and leave it unchanged in the z direction.
import antares
myt = antares.Treatment('scaling')
myt['base'] = base
myt['coordinates'] = ['x', 'y', 'z']
myt['factor'] = [2, 0.6, 1]
scaled_base = myt.execute()
Main functions¶
Example¶
The following examples creates a 2D structured base with a scalar variable and it uses the scaling treatment to scale the base by a factor of 0.5 in the x direction (scaling down) and by a factor of 2 in the y direction (scaling up).
import antares
import numpy as np
import os
output_folder = os.path.join("OUTPUT", "TreatmentScaling")
os.makedirs(output_folder, exist_ok=True)
X, Y = np.mgrid[0:10, 0:10]
# Create base to translate
base = antares.Base()
base.init()
base[0][0]['x'] = X
base[0][0]['y'] = Y
base[0][0]['myvar'] = X*Y # create a dummy variable
base.coordinate_names = ['x', 'y']
# Dump base to translate
w = antares.Writer('hdf_antares')
w['base'] = base
w['filename'] = os.path.join(output_folder, 'base_to_scale')
w.dump()
# Apply rotation treatment
scaling = antares.Treatment('scaling')
scaling['base'] = base
scaling['factor'] = [0.5, 2]
scaled_base = scaling.execute()
# Dump result
w = antares.Writer('hdf_antares')
w['base'] = scaled_base
w['filename'] = os.path.join(output_folder, 'scaled_base')
w.dump()
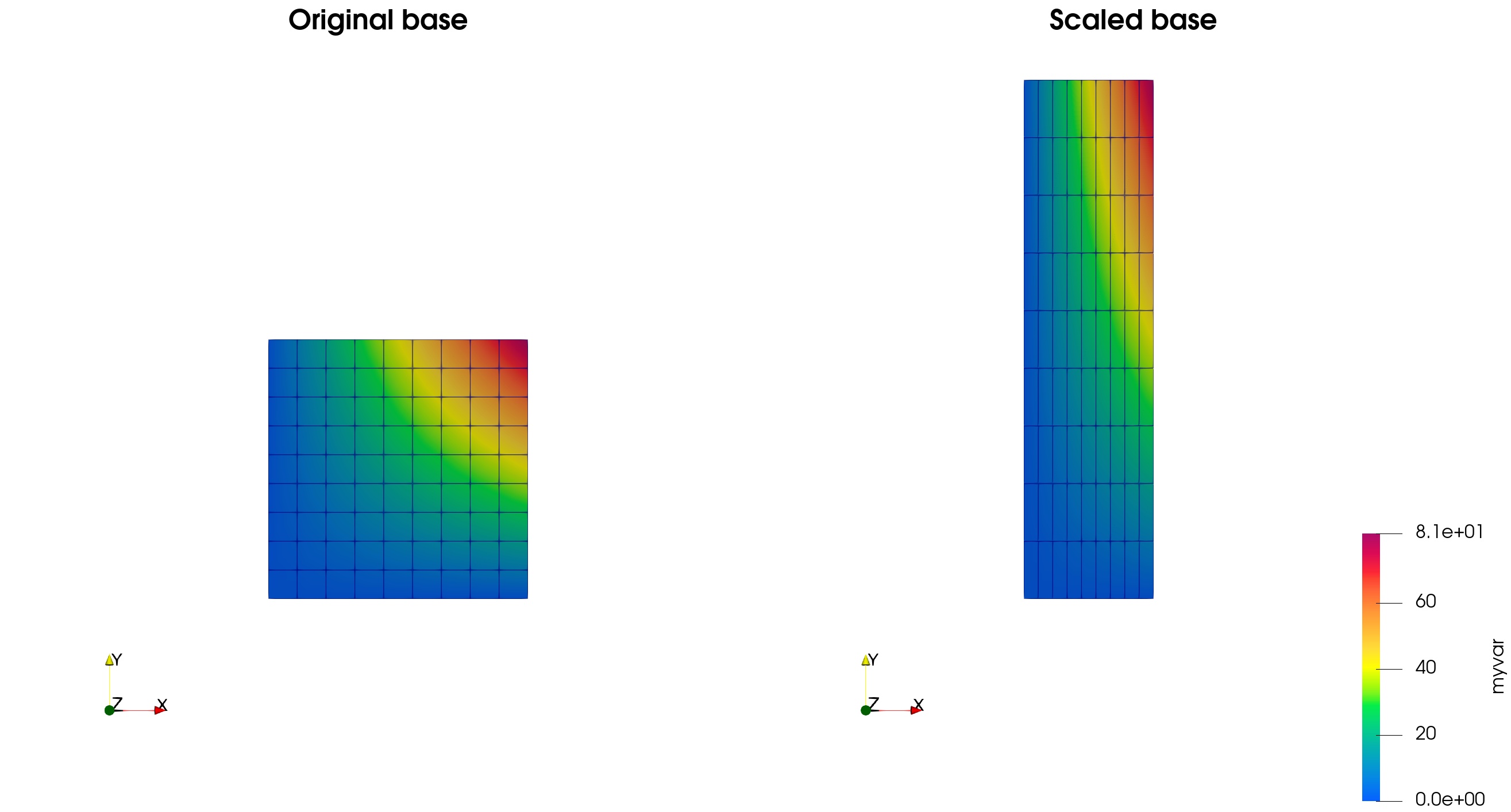